研究了一下,如何用ATmega 16单片机,来驱动字符型液晶显示芯片,现把研究心得写出来:
我手里的这个RT1601液晶显示模块,使用的是S6A0069显示芯片。
各个引脚简单说明一下:
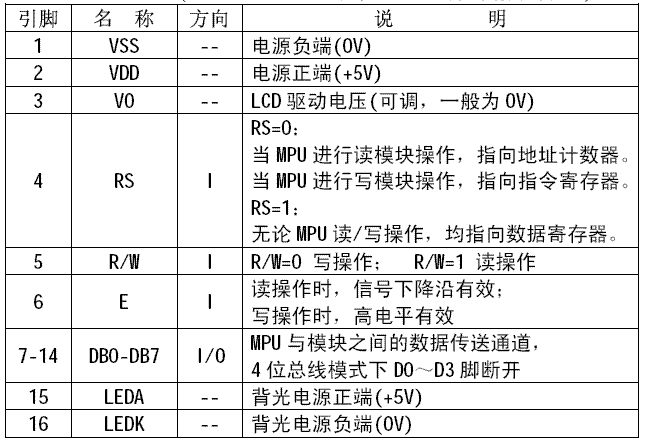
控制和数据引脚配置如下:
-------------------------------
PORTD_0 - RS
PORTD_1 - R/W
PORTD_2 - E
PORTA - DATA BUS
------------------------------
LCD.h
1
#ifndef _LCD_H_
2
#define_LCD_H_
3
/*****************************************
4
RS - PORTD_0
5
R/W - PORTD_1
6
E - PORTD_2
7
DB - PORTA
8
******************************************/
9
voidLCD_Set_RS(void);
10
voidLCD_Clear_RS(void);
11
voidLCD_Set_RW(void);
12
voidLCD_Clear_RW(void);
13
voidLCD_Set_E(void);
14
voidLCD_Clear_E(void);
15
voidLCD_CheckBF(void);
16
//Write Command
17
voidLCD_Write_Cmd(unsignedcharbyCmd);
18
//Write Data
19
voidLCD_Write_Data(unsignedcharbyData);
20
voidLCD_Delay_5ms(void);
21
voidLCD_Delay_500ms(void);
22
voidLCD_PortInit(void);
23
24
//Application Interface
25
voidLCD_Init(void);
26
voidLCD_Display(char*pstrText);
27
28
#endif
29
LCD.c
这里是液晶驱动的实现部分,对外开放两个接口:LCD_Init, LCD_Display。(我这个模块,是两行显示的,共16个字符)
1
#include
"LCD.h"2
#include<iom16v.h>
3
#include<macros.h>
4
5
/*****************************************
6
RS - PORTD_0
7
R/W - PORTD_1
8
E - PORTD_2
9
DB - PORTA
10
******************************************/
11
12
13
voidLCD_Set_RS(void)
14
{
15
PORTD|=(1<<0);
16
17
return;
18
}
19
20
voidLCD_Clear_RS(void)
21
{
22
PORTD&=~(1<<0);
23
24
return;
25
}
26
27
voidLCD_Set_RW(void)
28
{
29
PORTD|=(1<<1);
30
31
return;
32
}
33
34
voidLCD_Clear_RW(void)
35
{
36
PORTD&=~(1<<1);
37
38
return;
39
}
40
41
voidLCD_Set_E(void)
42
{
43
PORTD|=(1<<2);
44
45
return;
46
}
47
48
voidLCD_Clear_E(void)
49
{
50
PORTD&=~(1<<2);
51
52
return;
53
}
54
55
voidLCD_CheckBF(void)
56
{
57
DDRA=0x00;//Input
58
59
LCD_Clear_RS();
60
LCD_Set_RW();
61
LCD_Set_E();
62
while(PINA&0x80)
63
{
64
;
65
}
66
LCD_Clear_E();
67
68
DDRA=0xFF;//Output
69
70
return;
71
}
72
73
//Write Command
74
voidLCD_Write_Cmd(unsignedcharbyCmd)
75
{
76
LCD_CheckBF();
77
78
LCD_Clear_RS();
79
LCD_Clear_RW();
80
LCD_Set_E();
81
PORTA=byCmd;
82
LCD_Clear_E();
83
84
return;
85
}
86
87
//Write Data
88
voidLCD_Write_Data(unsignedcharbyData)
89
{
90
LCD_CheckBF();
91
92
LCD_Set_RS();
93
LCD_Clear_RW();
94
LCD_Set_E();
95
PORTA=byData;
96
LCD_Clear_E();
97
98
return;
99
}
100
101
voidLCD_Delay_5ms(void)
102
{
103
unsignedinti=5552;
104
while(i--)
105
{
106
;
107
}
108
109
return;
110
}
111
112
voidLCD_Delay_500ms(void)
113
{
114
unsignedchari=5;
115
unsignedcharj=0;
116
117
while(i--)
118
{
119
j=7269;
120
while(j--)
121
{
122
;
123
}
124
}
125
126
return;
127
}
128
129
//Port Init
130
voidLCD_PortInit(void)
131
{
132
PORTD=0x00;
133
DDRD=0xFF;//Output
134
DDRA=0xFF;
135
136
return;
137
}
138
139
//LCD Init
140
voidLCD_Init(void)
141
{
142
LCD_PortInit();
143
144
LCD_Delay_500ms();
145
146
LCD_Write_Cmd(0x38);//Function Set. 8bit data length, 2-line, 5*8 font
147
LCD_Delay_5ms();
148
LCD_Write_Cmd(0x0C);//Display ON/OFF Control. Display ON, Cursor OFF, Blink OFF
149
LCD_Delay_5ms();
150
LCD_Write_Cmd(0x01);//Display Clear.
151
LCD_Delay_5ms();
152
LCD_Write_Cmd(0x06);//Entry Mode Set. Increment mode, Entire shift off
153
154
return;
155
}
156
157
voidLCD_Display(char*pstrText)
158
{
159
unsignedchari=0;
160
161
LCD_Write_Cmd(0x80|0x00);//1 Line Position
162
while(*pstrText!=0x00)
163
{
164
if(i==8)
165
{
166
LCD_Write_Cmd(0x80|0x40);//2 Line Position
167
}
168
169
LCD_Write_Data(*(pstrText++));
170
i++;
171
}
172
173
return;
174
}
175
176
client端调用如下:
1
//ICC-AVR application builder : 2008-6-4 8:41:192
//Target : M163
//Crystal: 4.0000Mhz4
#include<iom16v.h>
5
#include<macros.h>
6
#include"LCD.h"
7
8
voidmain(void)
9
{
10
LCD_Init();
11
LCD_Display("RT1601Demo by WF");
12
13
while(1)
14
{
15
;
16
}
17
18
return;
19
}
好了,就这些,有关该芯片的详细资料,请查看 S6A0069资料。
好运!